Source code
A starter repo for FastAPI, using Fern
Latest version: 0.7.7
The FastAPI generator generates types and networking logic for your FastAPI server. This saves you time and add compile-time safety that you are serving the exact API that you specified in your API Definition.
Available on the open source plan.
What Fern generates
- Pydantic models for your API types
- Exceptions that you can throw for non-200 responses
- Abstract classes for you to define your business logic
- All the networking/HTTP logic to call your API
Adding the FastAPI generator
Example server
Venus is a FastAPI microservice in production that manages Fern’s auth (i.e. users, organizations, tokens).
Demo Video
Getting started
Let’s walk through the Fern FastAPI Starter.
Step 1: Define the API
Step 2: Run fern generate
This generates all the boilerplate code into generated/.
Step 3: Implement the server
Notice you only need to provide the business logic. Just implement the function, and Fern takes care of the rest.
And register your endpoints with Fern, which registers them with FastAPI under the hood:
Step 4: Compile
The type checker will warn you if you make mistakes implementing your API:
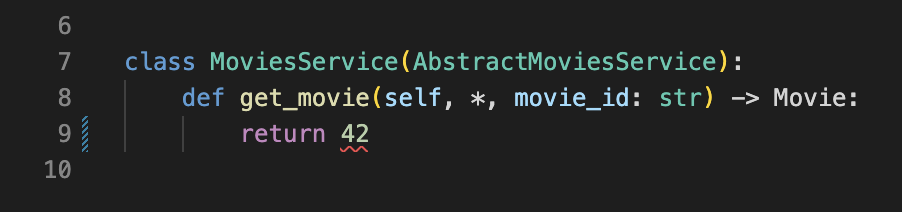
If you change the signature of the endpoint method, you’ll get an error:
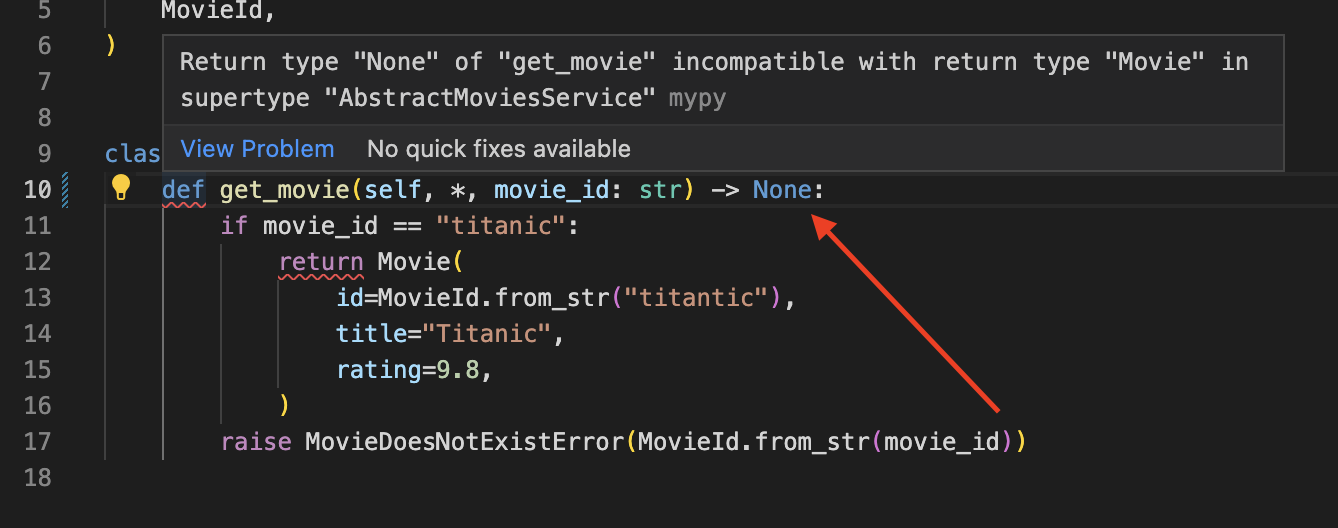
You can use the command line to check for compile errors: